Visual Studio Python Interpreter
There are dozens of Python development environments and ways to run a PyTorch program. Some common environments are Notepad with a command shell, IDLE, PyCharm, Visual Studio, and Visual Studio Code. My preferred approach is to use Notepad with a command shell as shown in Figure 1. Notepad has no learning curve and no hidden magic. Python is an interpreted language, and in order to run Python code and get Python IntelliSense, you must tell VS Code which interpreter to use. From within VS Code, select a Python 3 interpreter by opening the Command Palette (Ctrl+Shift+P), start typing the Python: Select Interpreter command to search, then select the command.
I’ve been doing some experimenting with pipenv to simplify my nascent Python programming workflows and also with Visual Studio Code as a cross-platform code editor. So naturally I want VS Code to use the python version from my pipenv-based virtual environment (as one does). I also want the compiled.pyc files to not show up in the explorer view. The same python interpreter is used for intellisense, autocomplete, linting, formatting, etc. (everything other than debugging). The standard interpreter used is the first “python” interpreter encountered in the current path.
This article originally appeared on Kite
Introduction to VS Code
Visual Studio Code Python Interpreter Path
Visual Studio Code (VS Code) is a source code editor developed by Microsoft that can run on Windows, macOS, and Linux. It is free, open-source, and is embedded with debugging tools, integrated terminals, built-in Git version control, code navigation, refactoring, and so on.
In addition to the built-in features, VS Code is highly customizable as users can install extensions to add additional support for languages, themes, and debuggers – among others.
Although VS Code comes with built-in support for JavaScript, TypeScript, and Node.js, it has a vibrant ecosystem of extensions for other languages including Python.
In this article, we’ll explore the vibrant support VS Code provides to make editing source code simpler for Python developers. We will walk through installing, browsing and adding extensions, as well as other useful packages to help with the Python development.
Initial Installation
Installing VS Code on MacOS
In this example, we’ll install the VS Code on macOS. Remember to install a Python distribution.
- Download VS Code
Open Chrome or your browser of choice, and head to the VS Code download section, and find the appropriate install for your platform (Windows, macOS, and Linux). - Begin Installation
Once the VS Code installation files have been downloaded, run the program and follow the instructions. Drag the program into applications, and verify the installation as requested. - Add to the Dock
If you want to add VS Code to the dock, right-click on the icon and select the option to keep it in the doc. - Launch from the command line
VS Code makes it super convenient for the developers to open the editor through the command line.- In your editor, click
COMMAND + SHIFT + P
to open the command palette - Type Shell and a list will appear
- Select the one that says:
Shell Command: Install code in PATH
- In your editor, click
Open your terminal, go to your preferred directory and type code
. (Notice the space between the word code and the period). It will open the folder in VS Code.
Installing VS Code on Windows
Installing on Windows is simply a matter of downloading the executable installer and running it as you would most other Windows applications.
- Download the Visual Studio Code installer for Windows.
- Once it is downloaded, run the installer (
VSCodeUserSetup-{version}.exe
). This will only take a minute. - By default, VS Code is installed under
C:users{username}AppDataLocalProgramsMicrosoft VS Code
Installing VS Code on Linux
Depending on your Flavor and preferences, there are a few different ways to install VS Code on Linux. Here are the three most popular:
- Package Manager (Beginners)
- Snap (Official)
- Apt (Ubuntu)
For Fedora, Redhat, and CentOS, check out the terminal instructions here.
Desktop Package Manager (Easiest for Beginners)
- Download the appropriate .deb or .rpm file from https://code.visualstudio.com/download
- Right-click and select “Install with Package Manager” depending on your Desktop Environment.
Snap (Official)
Snap is the “official” way to install VS Code from the terminal according to Microsoft.
Simply run:
$ sudo snap install --classic code
Note that you will need to have Snap installed on your system for this to work. You can also use code-insiders
in the command for VS Code Insider installation version.
Apt (Ubuntu)
Ubuntu users may wish to add the repository and install it with apt.
1. Add the Repository
$ curl https://packages.microsoft.com/keys/microsoft.asc | gpg --dearmor > microsoft.gpg
$ sudo install -o root -g root -m 644 microsoft.gpg /etc/apt/trusted.gpg.d/
$ sudo sh -c 'echo 'deb [arch=amd64] https://packages.microsoft.com/repos/vscode stable main' > /etc/apt/sources.list.d/vscode.list'
2. Update and install
$ sudo apt-get install apt-transport-https
$ sudo apt-get update
$ sudo apt-get install code # or code-insiders
The Python extension (all versions)
Before you can use Python with VS code, you’ll need to install the Python extension. The extension is published by Microsoft and can be found in the extension store by:
- Starting VS Code,
- Selecting Extensions in the menu panel on the far left,
- Searching for the Python extension from Microsoft and hitting ‘Install’.
Python interpreter selection
Now that we have VS Code installed with the Python plugin, let’s set our Python interpreter. Python is an interpreted language which means VS Code needs to know which interpreter to use to run our Python scripts.
In this article, we’ll be following steps for Python 3 so make sure you have Python 3 installed. If you want to continue with Python 2, the steps are relatively similar.
- In your editor, open the command palette by using the keyboard shortcut
COMMAND + SHIFT + P
- Search for Python: Select Interpreter
- Then select your Interpreter from the list
VS Code Python features
Python debugging:
One of the key features of VS Code is its debugging support. Automatic variable tracking, watch expressions, breakpoints, and call stack inspection are some of the debugging features VS Code provides.
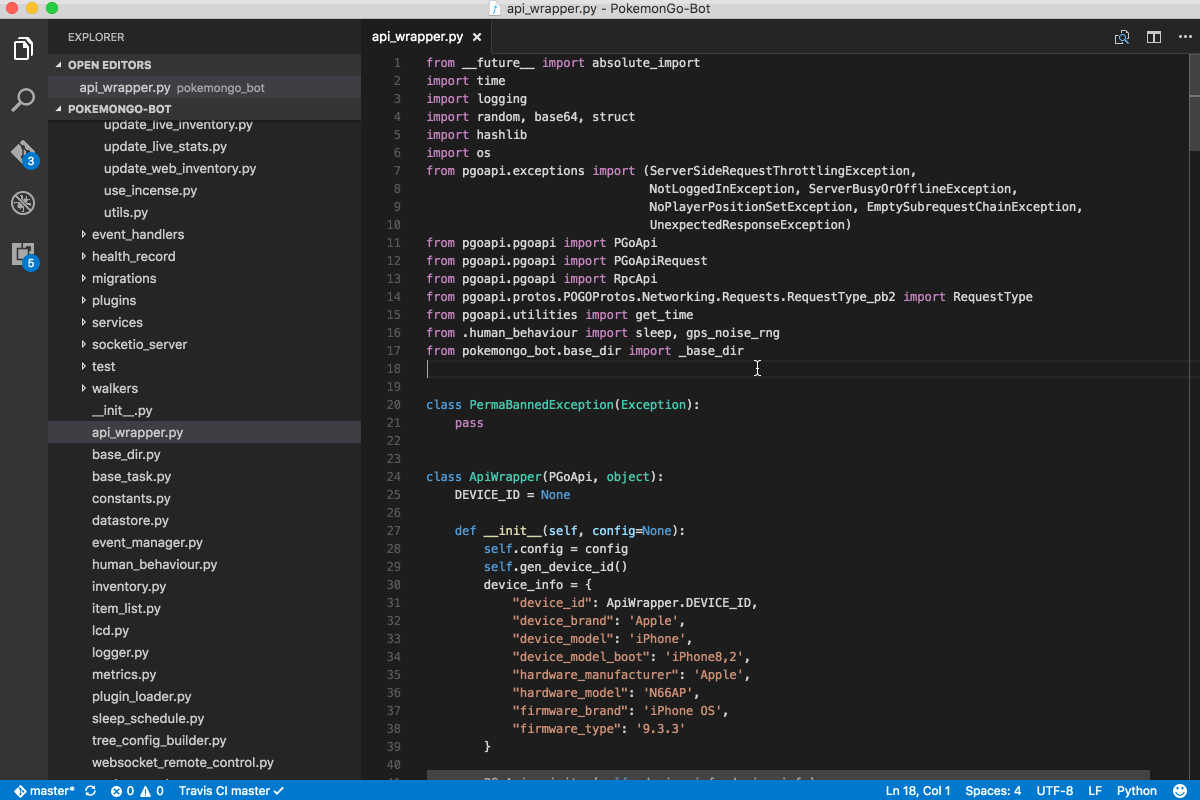
Debugging your current Python file is as simple as pressing F5
. As soon as the debugging session starts, a few things happen:
- The debug console panel appears with the commands that are being run and the output
- The debug view to the left of the editor with all the information related to debugging
- The debug toolbar appears along the top with debugging commands and configuration settings
The debug toolbar has the following commands from left to right: continue (F5
), step over (F10
), step into (F11
), step out (⇧F11
), restart (⇧⌘F5
), and stop (⇧F5
).
We can also toggle breakpoints by clicking on the editor margin or using F9
on the current line. When you set a breakpoint, a red circle appears in the gutter.
For more breakpoint control, we can edit the respective section in the Debug view.
For more complex projects, it’s helpful to configure and save debugging setup details. We can achieve this by creating a launch configuration file.
Creating a configuration file – launch.json – is pretty straightforward. From your project folder in VS Code, select the Configure gear icon on the Debug view top bar, and select Add Configuration to add Python.
Version control
VS Code has built-in support for source control management and includes Git support in-the-box. Additional SCM providers can be installed through extensions on the VS Code Marketplace.
The source control tab can be enabled by clicking the third icon in the activity bar or ⌃⇧G
. It shows the details of all the changes, staged changes and merge changes. Apart from displaying the repository changes, VS Code also offers you to commit files, clone repositories, push and pull changes, create and checkout branches, view and resolve merge conflicts, and view diffs.
Formatting
For programmers, readability is crucial, and code formatters make sure to improve the readability of your Python code. VS Code’s Python extension has default support for autopep8
.
Autopep8 is a tool that automatically formats Python code to conform to the PEP 8 style guide. PEP 8 has specific guidelines for line spacing, indents, spacing around operators, and so on. By following these conventions, autopep8
makes the code easier to comprehend.
To format a file use ⌃⇧F
or simply run the following command:
$ autopep8 --in-place --aggressive --aggressive <filename>
Where --in-place
makes changes to files in place and --aggressive
enables non-whitespace changes.
Run selection
There are many ways of running your script. But if you want to run a selected code or the code on the current line if there is no selection, SHIFT + ENTER
is a way to do it. It will run your code in the Python Terminal.
Alternatively, you can select Python: Run Selection/Line in Python Terminal
from the command palette to run the selected code.
Linting
The Python extension also comes with linting. As soon as you save the file, linting helps with analyzing the script for common syntactical, stylistic, and functional errors. It displays a list of all the issues found and depicts them visually with color squiggles in the code.
VS Code comes with the default linter – PyLint – but it can easily be customized in the command palette by selecting Python: Select Linter
.
Popular extensions:
VS Code is an excellent editor on its own, but you can increase its power through Extensions. Extensions let you customize and support your development workflow.
You can browse and install extensions from within VS Code. To bring up the Extensions view, click on the Extensions icon in the Activity Bar or use ⇧⌘X
. It will display a list of recommended as well as enabled extensions.
Click on the Install button and after a successful install, you’ll need to reload to enable the new extension.
Python
This is the official extension by Microsoft you installed earlier. It has rich support for all versions of Python. It includes features such as automatic indenting, linting, debugging, code navigation, code formatting, code definition, refactoring, unit tests, snippets, Python Interactive (Jupyter support), among others.
Kite
Kite is an AI-powered programming assistant that helps you write Python code inside Visual Studio Code. The Kite Engine needs to be installed in order for the extension to work properly. The extension itself provides the frontend that interfaces with the Kite Engine, which performs all the code analysis and machine learning.
Kite provides you with:
- Smart autocompletion powered by machine learning models trained on the entire open-source code universe
- Function signatures that show you the official signature of a function you’re currently using
- Instant documentation for the symbol underneath your cursor
autoDocstring
With more than eighty thousand downloads, autoDocstring by Nils Werner quickly generates docstrings for Python functions, chooses between several different types of docstring formats, and supports for args, kwargs, decorators, errors, and parameter types among other features.
Other popular plugins include Docker integration, Code Runner, Anaconda Extension Pack Trailing Spaces, and GitLens.
Getting the most from VS Code
VS Code is a powerful code editor and can be enhanced with the installation of a few extensions. If you want to get the best out of the VS Code, learn the ins and outs of the editor by going through the Interactive Playground. It provides a step-by-step guide to some of the advanced code editing features with interactive examples.
The following is only valid when the Python plugin is installed and enabled.
IntelliJ IDEA provides full integration with the Python interpreters running on remote hosts.
Before you start working with remote interpreters, make sure that the Vagrant and Docker plugin are installed and enabled. If the plugins are not activated, enable them on the Plugins page of the Settings / Preferences dialog as described in Manage plugins.
Depending on type of the remote interpreter, follow one of the procedures:
Ensure that the Python plugin is installed and enabled.
Navigate to File | Project StructureCtrl+Alt+Shift+S.
In the Project Structure dialog, select SDKs under the Platform Settings section, click , and from the popup menu, choose Python SDK.
In the left-hand pane of the Add Python Interpreter dialog, click SSH Interpreter.
In the right-hand pane select New server configuration, then specify server information (host, port, and username).
Alternatively, you can select Existing server configuration and choose any available deployment configuration from the list.
If needed, click to review the Connection settings, Mappings, and Excluded paths for the selected deployment configuration. Click Next to continue configuring an interpreter.
In the next dialog window, provide the authentication details to connect to the target server.
Select Password or Key pair (OpenSSL or PuTTY) and enter your password or passphrase.
Click Next to proceed with the final configuration step.
In the next dialog window, verify the path to the desired Python interpreter. You can accept default, or specify a different one. You have to configure the path mappings between your local project and the server. To do that, click next to the Sync folders field and enter the path to the local project folder and the path to the folder on the remote server.
You can also select the lowest checkbox to enable automatic upload of the local changes to the remote server.
Ensure that a Vagrant instance is created on your machine and properly initialized.
Ensure that the Python plugin is installed and enabled.
Navigate to File | Project StructureCtrl+Alt+Shift+S.
In the Project Structure dialog, select SDKs under the Platform Settings section, click , and from the popup menu, choose Python SDK.
In the left-hand pane of the Add Python Interpreter dialog, click Vagrant:
Click the browse button next to the field Vagrant instance folder, and specify the desired Vagrant instance folder.
This results in showing the link to Vagrant host URL.
The Python interpreter path field displays the path to the desired Python executable. You can accept default, or specify a different one.
Click OK. The configured remote interpreter is added to the list.
Click the Windows button in the lower-left corner of the screen and start typing
System Information
. To ensure that your system works well with WSL, upgrade your Windows to the latest available version.Install the Windows Subsystem for Linux and initialize your Linux distribution as described in the WSL Installation Guide.
Ensure that the Python plugin is installed and enabled.
Navigate to File | Project StructureCtrl+Alt+Shift+S.
In the Project Structure dialog, select SDKs under the Platform Settings section, click , and from the popup menu, choose Python SDK.
In the left-hand pane of the dialog, click WSL.
Select the Linux distribution and specify the path to the python executable in the selected Linux distribution. Typically, you should be looking for wsl.exe but you can specify any non default WSL distro.
Ensure that you have set up Docker on your machine.
Ensure that the Python plugin is installed and enabled.
Navigate to File | Project StructureCtrl+Alt+Shift+S.
In the Project Structure dialog, select SDKs under the Platform Settings section, click , and from the popup menu, choose Python SDK.
In the dialog that opens, select the Docker option, from the drop-down lists select the Docker server (if the server is missing, click New.. ), and specify the image name.
Python interpreter path should have the default value, for example,
python
:Click OK to complete the task.
Ensure that you have set up and run Docker Compose on your machine.
Ensure that the Python plugin is installed and enabled.
Navigate to File | Project StructureCtrl+Alt+Shift+S.
Wifi key finder hack wireless download. In the Project Structure dialog, select SDKs under the Platform Settings section, click , and from the popup menu, choose Python SDK.
In the dialog that opens, select the Docker Compose option, from the drop-down lists select the Docker server, Docker Compose service (here
web
), configuration file (heredocker-compose.yml
)and image name (herepython
).Next, wait while IntelliJ IDEA starts your Docker-Compose configuration to scan and index:
Click OK to complete the task.
Import Python Packages In Vs Code
For any of the configured Python interpreters (but Docker-based), you can:
